配置文件加密存储
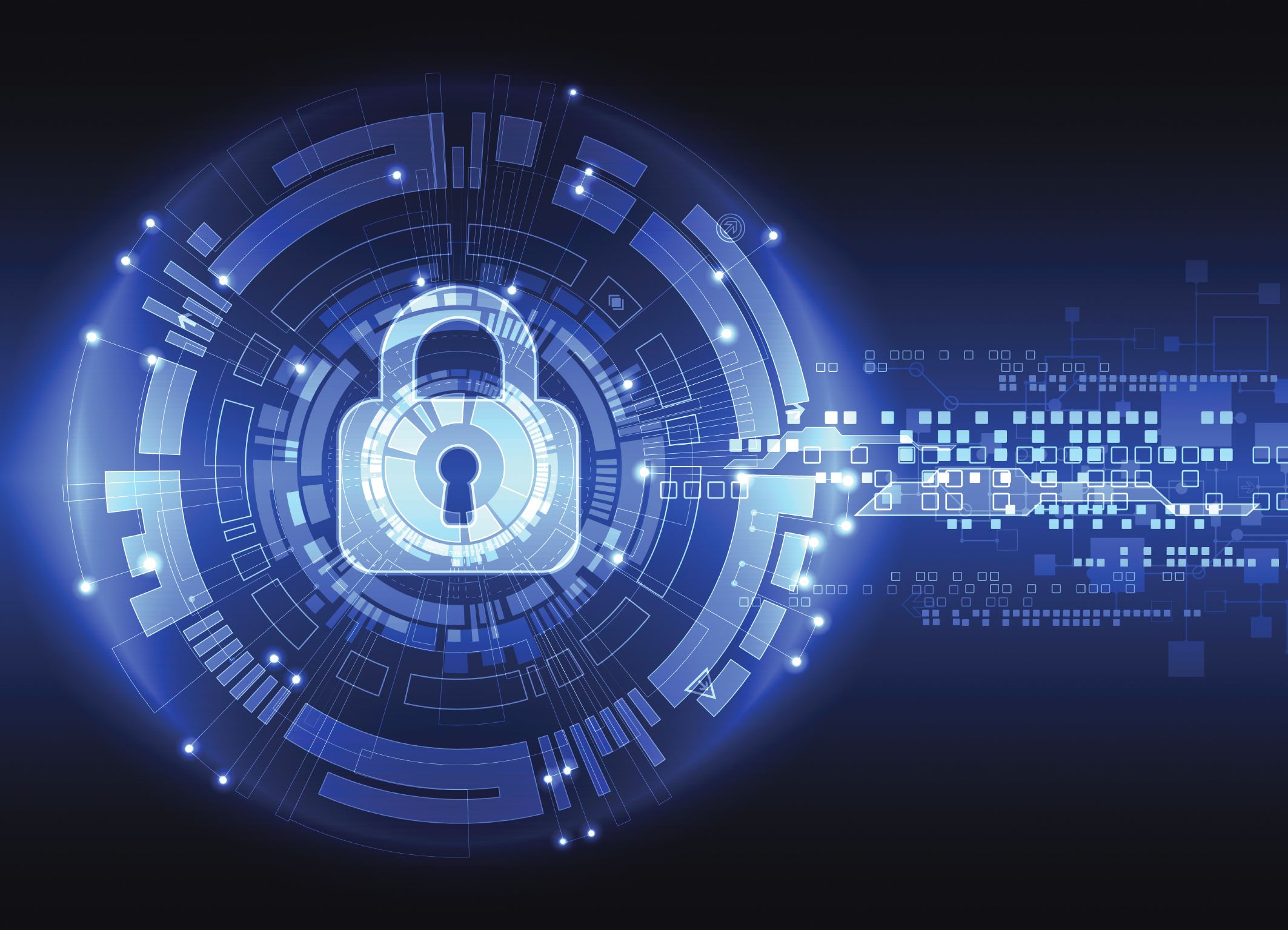
AI-摘要
GPT-4.0-turbo GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
配置文件加密存储
Takake1.导入jasypt坐标
添加jasypt依赖坐标
pom.xml
1 | <dependency> |
2.实现自定义配置文件加密算法
实现接口PBEStringEncryptor
com/gaomu/utils/crypto/SM4YmlEncryptor.java
1 | public class SM4YmlEncryptor implements PBEStringEncryptor { |
3.测试main方法
可在main方法中生成ENC密文
com/gaomu/utils/crypto/SM4YmlEncryptor.java
1 | public static void main(String[] args) { |
4.添加自定义加密算法配置
在springboot配置中注入Bean
com/gaomu/config/AppConfig.java
1 |
|
5.添加解密配置文件密钥
5.1.IDEA配置环境变量
如果是在IDEA中系统需要在IDEA启动配置中添加环境变量
添加如图添加环境变量配置
- 如果没有环境变量选项需要在修改选择中添加该选项
5.2.系统配置环境变量
- 如果是在Windows生产环境中需要在系统环境变量中配置
5.3.如果是在linux中配置环境变量
1 | # 添加环境变量 |
6.配置application.yml
使其生效还需要在springboot配置文件中配置
application.yml
1 | jasypt: |
7.配置加密信息
7.1.在main方法中生成数据库密码密文
7.2.在application.yml配置文件添加加密后的密文
- 密文需要使用ENC(cipher)标识,如此springboot启动配置才会解密该数据
1 | spring: |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果