基于某电力系统传输加解密操作BP插件编写
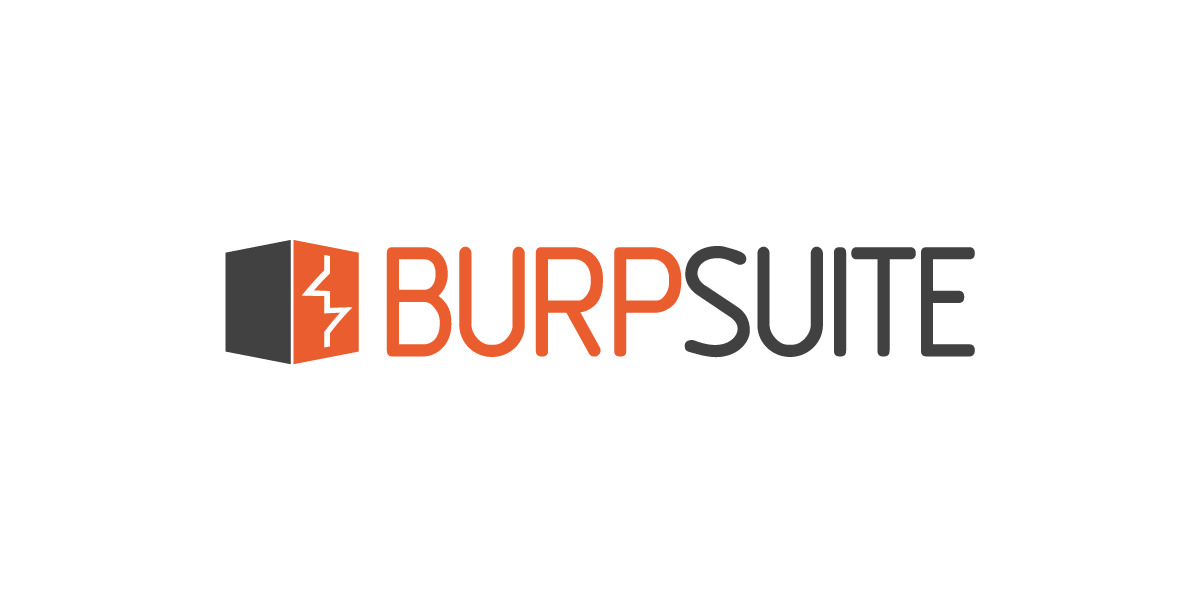
AI-摘要
GPT-4.0-turbo GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
基于某电力系统传输加解密操作BP插件编写
Takake1.WEB JS逆向出密钥和算法以及签名
- 通过前端JS逆向发现请求响应都使用的是SM4加密算法。且跟踪代码后发现密钥在前端进行了硬编码
- 而签名逻辑为url+body参数+‘-’+url参数+请求方法,最后替换掉斜线
2.复现完整加解密和签名逻辑
- 首先对加密数据进行BASE64解码为二进制数据,然后进行SM4ECB解密。加密就反过来即可。
- 签名逻辑复现
3.编写插件
3.1.新建IDEA Maven项目
- 我这里采用的是Maven项目,如果想用Groovy构建的也可以,Groovy构建的更快,官方也是用的Groovy,不过我用的不够熟悉,有时候构建容易出问题,所以我这采用Maven构建。需要注意的是JDK版本一定要是JAVA17版本以上的,因为我们这使用的是新版本的montoya-api,而不是老版本的API,所以必须使用高版本JAVA。
- pom文件配置
- 需要导入的是bp插件的API依赖两个,
1 |
|
- 下载BP新版插件示例 https://github.com/PortSwigger/burp-extensions-montoya-api-examples
- 下载burp-extension-input-editer和MyHttpHandler这两个代码示例
- 将示例中的JAVA代码均导入复制到我们的项目中。(以下几个文件)
- 然后将MyExtensionProvidedHttpRequestEditor和MyHttpRequestEditorProvider复制后重新更名为MyExtensionProvidedHttpResponseEditor和MyHttpResponseEditorProvider。
- 看名字就应该清楚,我们不仅需要对请求参数进行加解密还需要对响应也进行加解密,因此需要再拷贝一份。
3.2.编写加密解密函数
- 首先编写SM4解密函数,我是从我之前的模板整改的,为了编写代码更少。之后我也会将模板链接贴在下面,这是我写了很多套加解密逻辑最后形成的自己的模板,都是精华。
- 首先是对密钥key的处理,SM4密钥是我们从前端逆向出来的一个硬编码UTF8的数据。我为了方便直接使用CyberChef工具将这个密钥转换为hex字符串格式,大家也可以直接在代码里转换。无论密钥本身是什么格式的字符串,BASE64还是UTF8的数据转换为SM4的16进制密钥都是32个字符长度,因为SM4的密钥长度为128bit,所以如果不是这个长度那说明你的密钥是有问题的。
- 因为我们这获取到的密文是BASE64编码的所以需要对密文进行BASE64解码后再解密。
- 还有大家需要注意自己的填充模式,我这边使用的是无填充模式,通常SM4都会有填充的也就是PKCS5Padding。
- 为了方便,我将密钥KEY进行了硬编码,也就是根据代码逻辑中key我就不需要再传值了,之后调用函数的时候我直接传入空字符串就行了,如果需要传入不同的密钥再配置参数。
1 | public static String decryptECB(String cipherTxt, String key) { |
- 加密逻辑
1 | public static String encryptECB(String plainTxt, String key) { |
3.3.编写CustomRequestEditorTab文件
1 | public class CustomRequestEditorTab implements BurpExtension |
- 下图分别为插件名和日志输出。日志输出方式有很多中,具体大家可以看官方示例,我这只演示这一种。
3.4.请求处理器编写
- MyHttpRequestEditorProvider 不用做任何变动,保持和官方示例相同即可。
1 | class MyHttpRequestEditorProvider implements HttpRequestEditorProvider |
- MyExtensionProvidedHttpRequestEditor 编写
- MyExtensionProvidedHttpRequestEditor 初始化构造器
- getRequest 用于处理我们修改后的请求
- setRequestResponse 用于处理原始请求
- isEnabledFor 用于判断处理是否开启该处理器
- caption 返回该编辑器窗口的标签名字
- selectedData 用于判断请求是否有数据
- isModified 用于判断请求是否被修改过
1 | class MyExtensionProvidedHttpRequestEditor implements ExtensionProvidedHttpRequestEditor |
3.5.响应编辑处理器
- MyHttpResponseEditorProvider
1 | class MyHttpResponseEditorProvider implements HttpResponseEditorProvider |
- MyExtensionProvidedHttpResponseEditor, 和请求处理器类似
1 | class MyExtensionProvidedHttpResponseEditor implements ExtensionProvidedHttpResponseEditor |
3.6.伪造签名参数
- SM3签名
1 | public static String enSign(String data){ |
- MyHttpHandler
1 | class MyHttpHandler implements HttpHandler { |
3.7.打包-导入
- 清除-编译-打包
- 注意如果显示找不到主类,可进行如下配置
- 默认 点击确定即可
- 打包成功后会有两个jar包
- 导入插件
- 一定要选择包含依赖版本的jar包
- 导入成功后 会显示自己配置的日志输出。
4.总结
其实只要有固定的模板,代码编写还是比较简单的。
主要难在JS逆向部分,应用加密的不确定性,特别是同一权限集成系统之类的,代码量很大的话,我们很难定位到我们想要的位置,就无法逆向成功。
加解密BP插件模板下载地址,(个人模板),里面的加密编写的 都是国密的,如果要用其他密码加密算法,自己导入自己写就可以了,代码逻辑都是一样的。
MyHttpHandler 是很重要的,这个类可以用来对请求参数进行特定化修改,例如需要伪造签名,或者更新时间戳之类的,都需要用到这个类。还有就是可以给特定特征的请求添加笔记记录和颜色高亮显示等。
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果