JAVA常见编码转换
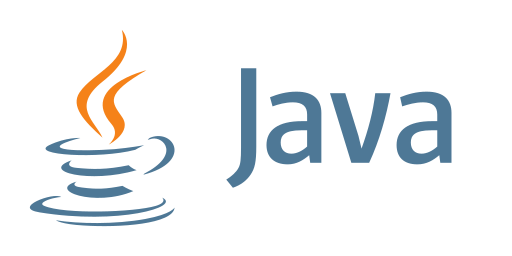
AI-摘要
GPT-4.0-turbo GPT
AI初始化中...
介绍自己 🙈
生成本文简介 👋
推荐相关文章 📖
前往主页 🏠
前往爱发电购买
JAVA常见编码转换
Takake1.UTF-8转换byte[]
1 | import java.nio.charset.StandardCharsets; |
- 前端部分
1 | let bufferKey = Buffer.from(key, "utf8") |
2.BASE64字符串转换byte[]
1 | import java.util.Base64; |
- 前端部分
1 | let bufferKey = Buffer.from(key, "base64") |
3.Hex转换byte[]
1 | //自实现 |
- 前端部分
1 | let bufferKey = Buffer.from(key, "hex") |
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果